目次
Migrating from Vuex to Pinia Guide
Introduction
Today, state management in the world of Vue.js has become an important topic for many developers. Vue.js provides various tools to efficiently handle data flow and state management. Among them, Vuex has long been loved by the Vue.js community as a state management library. However, a new state management library, Pinia, has emerged, opening up new possibilities for state management in Vue.js.
What is Pinia?
Pinia is a new library for simplifying and efficiently managing state in Vue.js applications. While inheriting the architecture of Vuex, Pinia provides a more simple and intuitive API, aiming to enhance developer productivity. Particularly, it has high compatibility with TypeScript, enabling the writing of more type-safe code.
Differences between Vuex and Pinia
There are several differences between Vuex and Pinia. Vuex is the official state management library for Vue.js, offering abundant features and community support. On the other hand, Pinia is a newer library, requiring simpler API and setup compared to Vuex. Additionally, Pinia has better compatibility with TypeScript, making some tasks that were difficult in Vuex easier to accomplish.
Advantages of Pinia
The main advantages of Pinia are its simplicity and compatibility with TypeScript. This allows developers to manage the state of applications more quickly and efficiently. Furthermore, Pinia provides a more modern API compared to Vuex, enabling developers to easily implement state management best practices. Additionally, Pinia simplifies the configuration and management of state management, making it quickly adoptable in new projects.
In this article, we will explain the process of migrating from Vuex to Pinia step by step. Let’s learn how to efficiently migrate from the existing setup of Vuex to the new setup of Pinia.
Preparation before migrating to Pinia
In this section, we will describe the tools and environment setup needed before migrating to Pinia, as well as checking the current status of Vuex. These steps are important to ensure a smooth migration.
Setup of necessary tools and environment
Before starting the migration to Pinia, you need to set up several tools and environments. First, install Node.js and npm, and set up your Vue.js project. Next, install Vuex and check your current Vuex store setup.
- Installation of Node.js and npm:
- Download and install from the official Node.js website.
- Once the installation is complete, execute
node -v
andnpm -v
in the terminal to confirm that they have been installed correctly.
- Setting Up a Vue.js Project:
- In the terminal, execute
vue create my-vue-project
to create a new Vue.js project. - Move to the project directory by executing
cd my-vue-project
.
- Installing Vuex:
- Execute
npm install vuex --save
to install Vuex in your project.
With these steps, the basic setup is complete.
Checking The Current State of Vuex
It’s important to check the current state of your Vuex store before transitioning to Pinia. Follow the steps below:
- Confirm the Structure of Your Vuex Store:
- Examine the source code to understand the current structure of your Vuex store.
- Check the store’s modules, actions, mutations, and getters.
- Understanding Vuex Store Data Flow:
- Refer to the Vuex official documentation to understand the data flow.
Through these checks, you can gather the necessary information for transitioning to Pinia. The next section explains the actual process of transitioning to Pinia.
Installing and Configuring Pinia
When considering transitioning the state management in Vue.js, the first step is to install and configure Pinia. This section explains the installation of Pinia, basic settings, and how to create and use a store.
Installing Pinia
Installing Pinia is incredibly straightforward. You can accomplish it easily using either npm or Yarn. Run the following command in your project’s root directory to install Pinia.
npm install pinia
or
yarn add pinia
Setting up main.ts
Once the installation is complete, you’ll need to configure your main.ts file next. In this file, you create an instance of Pinia and inject it into your Vue application.
// main.ts
import { createApp } from 'vue';
import { createPinia } from 'pinia';
import App from './App.vue';
const app = createApp(App);
const pinia = createPinia();
app.use(pinia);
app.mount('#app');
Creating a Pinia Store
Creating a Pinia store is very similar to creating a Vuex store, but it’s more straightforward and intuitive. Here’s how to create a new store:
// stores/counter.js
import { defineStore } from 'pinia';
export const useCounterStore = defineStore({
id: 'counter',
state: () => ({
count: 0
}),
actions: {
increment() {
this.count++;
}
}
});
Using the Store
The created Pinia store can be easily used within Vue components. In the following example, we are creating a button to increment the counter.
\`\`\`
// components/Counter.vue
<template>
<button @click="increment">{{ count }}</button>
</template>
<script>
import { useCounterStore } from '@/stores/counter';
export default {
setup() {
const counter = useCounterStore();
return {
count: counter.count,
increment: counter.increment
};
}
}
</script>
\`\`\`
Thus, Pinia provides a simpler and more intuitive API compared to Vuex, making the setup and usage of state management straightforward. The next section will provide a detailed explanation on migrating code from Vuex to Pinia.
Migrating Code from Vuex to Pinia
Pinia is a new state management library as an alternative to Vuex. Here, we will explain the basic migration methods from Vuex to Pinia, along with specific code examples.
Setting Up on the Store Side
Unlike the setup in Vuex, Pinia offers a concise and intuitive setup method.
JavaScript
\`\`\`
// Vuex setup
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex);
export default new Vuex.Store({
state: {
count: 0
},
mutations: {
increment(state) {
state.count++;
}
}
});
// Pinia setup
import { createPinia } from 'pinia';
const pinia = createPinia();
export const useStore = () => {
return {
state: ref({ count: 0 }),
increment() {
this.state.count++;
}
}
};
\`\`\`
Handling Removed Mutations
With Pinia, there’s no need to define mutations like in Vuex. Instead, you can define methods directly to change the state.
Updating Components
Lastly, we will discuss the method of updating on the component side. Unlike Vuex, Pinia uses the Composition API to manage state within components.
\`\`\`
// Component using Vuex
import { mapState } from 'vuex';
export default {
computed: {
...mapState(['count'])
},
methods: {
increment() {
this.$store.commit('increment');
}
}
}
// Component using Pinia
import { useStore } from '../stores/store';
export default {
setup() {
const store = useStore();
return {
count: store.state.count,
increment: store.increment
};
}
}
\`\`\`
Refer to this migration guide to efficiently manage state in your Vue.js projects.
Migrating from Vuex to Pinia: Summary and Points to Note
Migrating from Vuex to Pinia might come with some challenges depending on the scale and complexity of the project. However, with proper preparation and understanding, a smooth migration can be achieved.
Possible Issues and Solutions Encountered During Migration
During the migration process, especially when certain features or settings in Vuex do not directly map to Pinia, issues may arise. The official Pinia documentation provides detailed guidance on migrating from Vuex to Pinia.
Best Practices for Pinia Migration
- Incremental Migration: For large-scale projects, it is recommended to start migrating your Vuex store to Pinia gradually, rather than doing it all at once.
- Testing: It is crucial to perform sufficient testing at each stage of the migration to ensure that the application is functioning correctly.
- Community Support: The Pinia community is vibrant and offers support through GitHub and Discord.
Additional Resources and Support
The Pinia community is active, providing a wealth of resources and support. Additionally, the official documentation contains detailed information on migrating from Vuex to Pinia.
Conclusion
The landscape of state management in Vue.js is continually evolving, and Pinia is a prime example of this evolution. Pinia’s simplicity and efficiency offer new options and possibilities to the Vue.js community.
Pinia Community and Support
The Pinia community is active on GitHub and Discord, providing support for any questions or issues you may have. Additionally, tutorials and guides provided by the community and the official documentation aid in learning and adapting to Pinia.
Future Trends in Vue.js State Management
Pinia is one of many projects shaping the future of state management in Vue.js. The library’s development is a positive step for the Vue.js community. For developers seeking the optimal method for state management, Pinia is definitely worth considering.
Learn Vue.js with Skilled
Skilled is a specialized, practical programming learning service focusing on frontend development.
We cover everything from coding to becoming a frontend engineer, providing a service where you can acquire not just knowledge but actual “skills” through practical learning.
We are currently running a free trial campaign, so if you’re interested, please take advantage of the free trial.
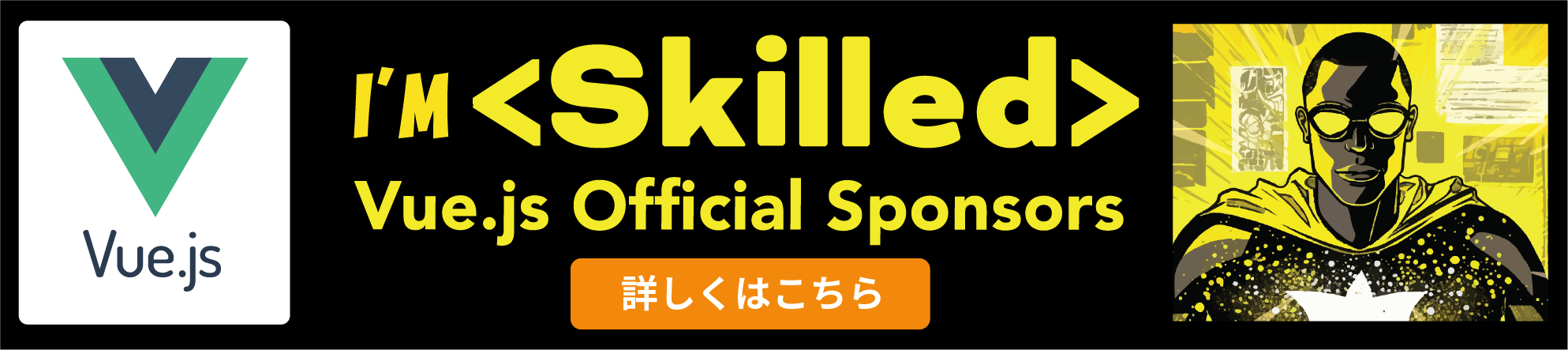